People love to throw around the idea that you can “master” JavaScript in a year. But what does that really mean? Is it just understanding the basics, or could you build a slick web app on your own? You’re probably wondering if you need to eat, sleep, and breathe code for twelve months straight—or if you can squeeze it in after work and still get pretty far.
Let's make one thing clear. Learning JavaScript is not just about memorizing syntax. It's about building stuff—real stuff that works, breaks, and teaches you along the way. You’ll run into weird bugs, unclear docs, and enough Stack Overflow tabs to make your head spin. But every line of code you write gets you a step closer to actually getting it.
The shortcuts? There aren’t any silver bullets, but there are smart ways to learn so you don’t waste time. For example, spending an extra hour practicing with small projects beats rereading a tutorial for the tenth time. And you don’t need to know everything before you start showing your work—even the pros Google stuff daily.
- The One-Year Mastery Myth
- The Core Skills You Need
- Common Pitfalls (and How to Dodge Them)
- A Realistic Roadmap
- Speed Boosters: Habits and Hacks
- What ‘Mastery’ Actually Looks Like
The One-Year Mastery Myth
The internet is full of claims like, “Become a JavaScript master in twelve months!” But the word “master” gets tossed around way too loosely. Here’s the hard truth: nobody learns everything about JavaScript in a year. Even seasoned developers with a decade of experience still hit problems that make them scratch their heads.
That said, you can absolutely get really good at JavaScript—enough to build real projects, snag a job, or even freelance—within a year if you focus and use the right learning approach. The key is to define what “mastery” means for you. Are you aiming to build interactive websites, work as a front-end developer, or crack interviews for big tech companies? Each goal has a different bar for what’s considered mastery.
To get a clear perspective, let’s look at what’s actually required for different kinds of JavaScript roles:
Role/Goal | Skill Level Needed After 1 Year | Possible? |
---|---|---|
Front-End Web Developer | Strong understanding of core concepts and frameworks | Yes, with daily practice |
Back-End (Node.js) Developer | Good grasp of JavaScript fundamentals plus server-side basics | Challenging, but possible with focus |
JavaScript Expert | Deep understanding of every quirk and advanced topic (memory management, performance optimizations, engine internals) | No, takes several years |
Learning speed depends on a ton of factors: your background, time commitment, and whether you use proven resources—like interactive coding platforms or reputable courses. According to a Stack Overflow 2023 survey, the majority of professional developers say they started feeling "confident" with JavaScript after about 1-2 years of active use. That’s people coding, breaking, and fixing stuff for at least several hours every week.
The myth comes from a bad habit—thinking that going through one course, or reading one book, means you've “mastered” the language. In reality, mastery is all about how you apply the stuff you know, not how many hours you’ve watched tutorials. If one-year mastery means you can confidently build things, debug, and adapt when new features roll out, then yes—you’re on the right track. But expecting to know every corner of JavaScript? That’s not how it works, not even for the pros.
The Core Skills You Need
If you want to get serious with JavaScript, you need to know beyond just how to declare a variable or write an if statement. The language is deeper than what most beginner courses cover. So, what actually counts as the "core" skills?
First, get solid with the basics. Stuff like data types (numbers, strings, booleans), arrays, objects, functions, and loops comes up everywhere. You’ll also need to really understand how the Document Object Model (DOM) works—because almost every interactive website relies on that. Don’t skip this, even if it’s boring at first.
Scope, closures, callbacks, and promises are things that usually trip people up. You can’t ignore them if you’re aiming for anything beyond a basic script. JavaScript wouldn’t be JavaScript without async code, and you’ll want to get familiar with async/await so your apps don’t freeze up when talking to APIs.
Speaking of APIs, actually using them—fetching data, sending requests, and handling responses—is where the rubber meets the road. That’s how sites show live updates or map your location in real-time.
- Variables, data types, and operators
- Functions (arrow vs. regular), parameters, return values
- Arrays, objects, and common array/object methods (map, filter, reduce, etc.)
- DOM manipulation and event handling
- ES6 features (let/const, template literals, destructuring, spread/rest)
- Asynchronous JavaScript (callbacks, promises, async/await, fetch API)
- Basic debugging with console and browser dev tools
- Understanding of modules and how to organize code
If you’ve ever wondered what areas most beginners get stuck on, check this out:
Skill | Difficulty (1-5) | Common Struggle |
---|---|---|
Variables & Data Types | 1 | Simple, but typos break things |
Functions | 2 | Forgetting to return values |
DOM Manipulation | 3 | Not finding the right element |
Async/Await | 4 | Mixing callbacks and promises |
Closures & Scope | 5 | Variables not behaving as expected |
You don’t need to master everything right out the gate. Start with the basics, then tackle these trickier concepts as you run into them in real projects. The point: don’t just read about these topics. Code them, break them, and see what happens. That’s how you really lock in the core skills you’ll need this year.
Common Pitfalls (and How to Dodge Them)
The road to mastering JavaScript is packed with traps that catch both beginners and folks with some coding under their belt. Let’s shine a light on the big ones, so you don’t end up spinning your wheels.
One of the first mistakes is skipping the basics. It’s tempting to jump into frameworks like React right away, but without knowing core JavaScript—things like how functions work, what 'this' actually means, or how scopes and closures mess with your variables—you’ll get stuck fast. You need a strong foundation, or the house falls down.
Tutorial hell is another classic pitfall. You start with one course, then jump to another, then a YouTube playlist, and before you know it, you’ve watched a hundred hours but barely written any code yourself. Passive learning can make you think you’re making progress, but hands-on projects are where real growth happens. If you’re not actually building stuff, you’re just collecting trivia.
Copy-pasting code without understanding is a huge time-waster. It might get your app working for now, but when something breaks (and it will), you won’t know what went wrong. Always take a few minutes to break apart someone else’s code and figure out what it’s doing before dropping it into your project.
Many people also ignore errors and warnings. JavaScript will often tell you exactly what went wrong, but it’s easy to brush those messages aside in a rush. Learning to read and understand those errors can save hours of frustration. Don’t just Google the message—try to explain it to yourself first, and then look up the parts you’re missing.
Here’s how to sidestep the biggest pitfalls:
- Stick to one main learning path for a while (one book, course, or structured resource).
- Code every single day, even if it's just 20 minutes. Consistency beats long, one-off sessions.
- Write code from scratch instead of copy-pasting—practice typing out examples and tweaking them.
- Break big projects into small tasks. This makes it less likely you’ll get overwhelmed and quit.
- Get comfortable with reading documentation, not just tutorials. Docs might look scary, but they’re how pros learn new features the fastest.
- Ask questions when you’re stuck, but try to explain your problem out loud first—sometimes, the answer pops out just by talking it through.
Avoiding these common mistakes puts you way ahead. After all, the people who stick with it and keep practicing the right way are the ones who actually master it—even in a year.
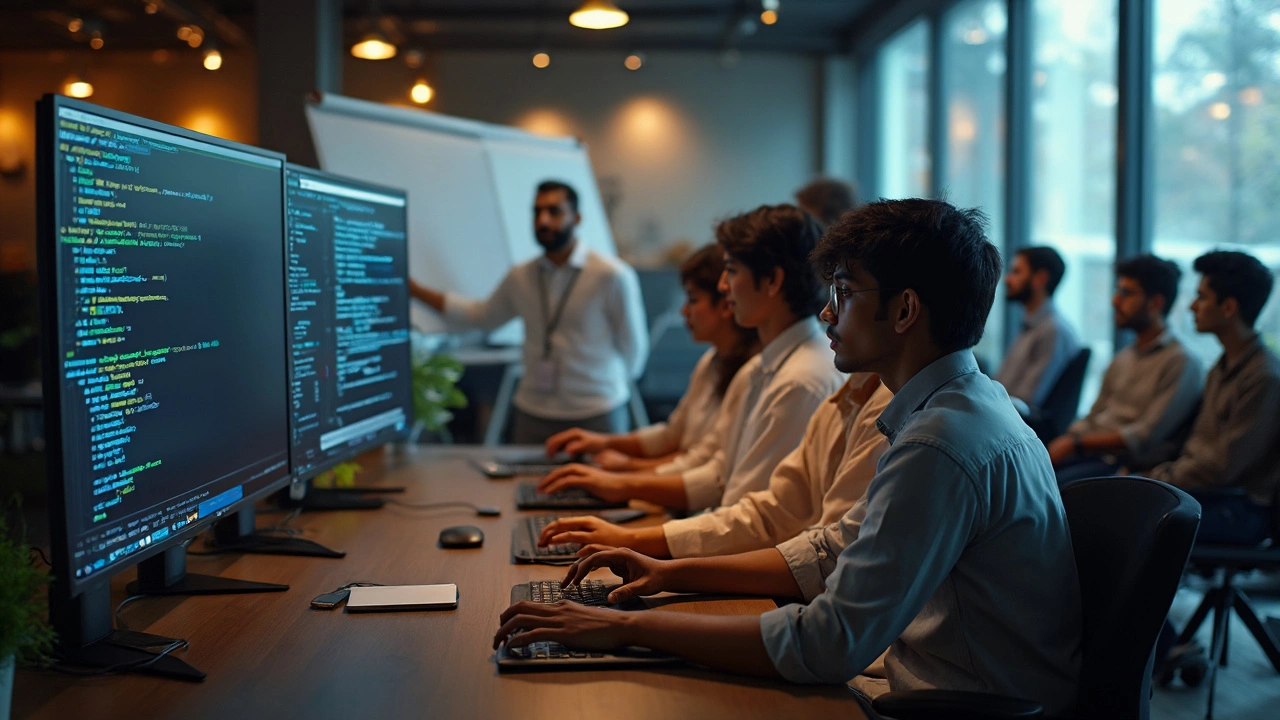
A Realistic Roadmap
So, how do you actually go from zero to solid in JavaScript within a year? Here’s the thing: you need structure, focus, and the right projects at the right time. One of the biggest mistakes? Jumping from topic to topic without getting comfortable with the basics first.
A smart first step is to set goals for every month. Here’s a straight-shooting example of what a year-long plan might look like. You don’t have to follow it exactly, but having milestones helps you measure real progress:
Month(s) | Main Focus | Output/Skills |
---|---|---|
1-2 | Basics: Variables, functions, data types, loops, conditionals | Simple scripts, console apps |
3-4 | DOM manipulation & events | Add interactivity to pages |
5-6 | APIs & asynchronous JS (fetch, promises, async/await) | Build simple apps that call external APIs |
7-8 | Frameworks: Intro to React or Vue | Basic single-page apps, learn component logic |
9-10 | Testing, debugging, and tooling (npm, webpack, linters) | Write cleaner, more reliable code |
11-12 | Personal projects & portfolio | Showcase apps, version control (Git), deployment |
Notice how you move from simple scripts to hands-on projects and frameworks. It’s not about speed-running tutorials. The sweet spot is steady, practical building—each month, try to finish at least one hands-on thing you can actually demo.
To nail down the basics, popular courses like freeCodeCamp or The Odin Project can take you from “I dunno what a variable is” to building stuff in a browser. Video tutorials, like Traversy Media or The Net Ninja on YouTube, break down tricky concepts in plain English. Stack Overflow’s 2023 survey said over 70% of working developers use online coding platforms for regular practice—so make that part of your routine, too.
Don’t forget to actually deploy your work. Even if it’s a tiny calculator or to-do list, put it on GitHub or Netlify. Real employers care about seeing working code, not just pretty résumés.
- Review your progress every month and ask yourself: “What can I build now that I couldn’t last month?”
- Mix up your learning: read, watch, code, and build.
- If you get stuck, focus on small wins and revisit tough spots after a break.
Consistency matters way more than marathon binge-coding. 5-10 focused hours a week can add up to crazy results in a year, especially when you’re putting what you learn into practice right away.
Speed Boosters: Habits and Hacks
If you're serious about learning JavaScript, it helps to stack the deck in your favor. Some habits will save you months, while a few mistakes can drag you back to square one. Here’s what really works for getting good, fast.
- Code every day. Even 20 minutes counts. It sounds cliché, but brains love repetition. The difference between coding “whenever” and building a daily streak is night and day—apps like GitHub even track this, and it’s fun to keep your streak alive.
- Write, don’t just watch or read. Tutorials feel safe, but you won’t really get it until your own code falls apart and you have to fix it. After learning a new thing, type it out yourself, break it, and mess with it.
- Mix up projects. Cloning a to-do list app is fine, but after your second one, move on. Try a weather app, a little game, or a website that uses an API. You’ll bump into new ways of thinking, and your skills will stack up faster.
- Ask questions right away. Google, ChatGPT, forums, a friend—just don’t let yourself get stuck for hours. Some pros recommend the ‘15-minute rule’: if you’re stuck for 15 minutes, look for help. This keeps you moving and learning new stuff.
- Get real feedback. Don’t code in a bubble. Post your code on GitHub, join a Discord server, or ask for reviews on Reddit. Even one comment can shine a light on sloppy habits or cool tricks you missed.
- Track what you learn. Keep a simple journal, log, or note. Every time you solve a weird bug or figure out a new concept, jot it down. Reading these back gives you a confidence boost and helps spot gaps.
The fastest learners treat learning like a game. They celebrate tiny wins, like fixing a bug or finishing a tiny project. Small progress adds up, and honestly, most of what you build in the first year will be forgettable—but the lessons won’t be. The people who keep going are the ones who find ways to have fun with it and don’t let one bug kill their momentum.
What ‘Mastery’ Actually Looks Like
People throw the word “mastery” around way too loosely, especially when it comes to JavaScript. The truth? Mastery isn’t about knowing every quirk or being able to recite method names from memory. It's about solving real problems, fast, and writing code that others can actually read and maintain. When you hit that point where you can jump into any project, spot issues quick, and build what you imagine without constantly running to Google for the basics, you’re on the right track.
Most hiring managers aren’t expecting someone with one year of experience to be an expert. But there are signs you’re becoming really good at JavaScript:
- You can build interactive apps from scratch without relying on copy-paste code.
- Your code is clean, organized, and you actually understand why it works.
- You can spot bugs early and know where to look when things break.
- You’re comfortable with async concepts, like promises and async/await, and don’t panic when you see them in code.
- You’ve worked with at least one big JavaScript framework (React, Vue, or Angular) and know how to debug real issues.
You don’t need to have created the next Facebook, but you should have a few solid projects you’re proud of—something you can show and explain in detail.
According to Dan Abramov, one of the minds behind React, true mastery is about mindset, not magic:
“Mastery is not about knowing everything, but knowing how to find what you need when you need it—and never being afraid to ask questions.”
The best JavaScript devs stay curious. They never stop learning, they read other people’s code, and they aren’t embarrassed to admit when they don’t know something. Real mastery is more about momentum than a finish line. You’ll always find a new technique, pattern, or bug that keeps you on your toes. But if you’re building, learning from your mistakes, and helping others do the same, that’s what being a ‘JavaScript master’ actually looks like today.